QA testers everywhere are trapped in a tedious cycle of manual testing, feeling like there’s no escape. But hold on a second – imagine yourself in Sarah’s shoes, executing hundreds of test cases in minutes with just a single command.
Picture this: It’s 2 AM, and Sarah, a QA tester at a bustling tech startup, is burning the midnight oil. She’s manually clicking through endless test scenarios, her eyes glazed over from staring at the screen. “There has to be a better way,” she mutters, rubbing her tired eyes.
Fast forward six months. Sarah strolls into the office at 9 AM, coffee in hand, wearing a confident smile. She opens her laptop, runs a command, and watches as hundreds of test cases execute flawlessly in minutes. Her colleagues gather around, amazed at the efficiency. “How did you do that?” they ask in awe.
Sarah’s secret? She mastered Java for QA automation.
This isn’t just Sarah’s story. It’s a transformative journey that countless QA professionals are embarking on. In the current climate, manual testing is no longer enough. Companies are hungry for QA engineers who can automate, streamline, and revolutionize their testing processes.
Java, the powerhouse of software development, is your ticket to success in the world of quality assurance. It’s the key that unlocked Sarah’s potential, and it can do the same for you. Whether you’re a beginner feeling overwhelmed by coding or an experienced tester looking to level up, this guide is your roadmap to Java mastery.
How did she do it? By mastering Java for QA automation, and you can do the same with the help of this guide.
1. Why Java for QA Automation?
Before we jump into the learning strategies, let’s address the elephant in the room: Why Java?
Java’s popularity in QA automation is no accident. Its robust ecosystem, platform independence, and extensive libraries make it a go-to choice for test automation frameworks. According to the 2023 Stack Overflow Developer Survey, Java remains in the top 10 most popular programming languages, with 30.49% of developers using it regularly.
For QA engineers, Java offers:
– Selenium WebDriver support for web application testing
– REST Assured for API testing
– TestNG and JUnit for test management
– Jenkins integration for continuous integration and delivery
Choosing Java for our QA automation framework was a great addition to our tech stack. Its robust ecosystem and extensive libraries allowed us to build a scalable, maintainable test suite that cut our release cycle time by 40%. Java’s learning curve may be steep, but the long-term benefits for our team have been immeasurable.
Now that we’ve established Java’s importance, let’s explore how to master it.
2. Start with the Basics: Java Fundamentals
Every journey begins with a single step, and your Java adventure is no exception. Begin by mastering the fundamentals:
– Variables and data types
– Control structures (if-else, loops)
– Object-oriented programming concepts
– Exception handling
Code example
public class QAAutomationBasics {
public static void main(String[] args) {
// Variables and data types
String testName = "Login Test";
boolean testPassed = true;
int numberOfAttempts = 3;
// Control structures
if (testPassed) {
System.out.println(testName + " passed successfully!");
} else {
System.out.println(testName + " failed after " + numberOfAttempts + " attempts.");
}
// Loop
for (int i = 1; i <= numberOfAttempts; i++) {
System.out.println("Attempt " + i);
}
// Exception handling
try {
// Simulating a test step
performTestStep();
} catch (Exception e) {
System.out.println("Test step failed: " + e.getMessage());
}
}
private static void performTestStep() throws Exception {
// Simulating a test step that might throw an exception
double randomValue = Math.random();
if (randomValue < 0.5) {
throw new Exception("Random failure occurred");
}
}
}
This Java code defines a class named QAAutomationBasics that simulates basic concepts of QA (Quality Assurance) automation testing. Let’s break down the code step-by-step:
Class Definition
public class QAAutomationBasics {
- The QAAutomationBasics class is a public class, meaning it can be accessed from other classes.
Main Method
public static void main(String[] args) {
- The main method is the entry point of the program. The public keyword allows it to be called from outside the class, static means it belongs to the class rather than an instance of the class, and void means it does not return a value.
Variables and Data Types
// Variables and data types
String testName = "Login Test";
boolean testPassed = true;
int numberOfAttempts = 3;
- String testName initializes a string variable with the name of the test.
- boolean testPassed initializes a boolean variable to indicate whether the test passed or not.
- int numberOfAttempts initializes an integer variable to store the number of attempts for the test.
Control Structures
// Control structures
if (testPassed) {
System.out.println(testName + " passed successfully!");
} else {
System.out.println(testName + " failed after " + numberOfAttempts + " attempts.");
}
- The if statement checks if the test passed. If testPassed is true, it prints that the test passed successfully. If false, it prints that the test failed after a certain number of attempts.
Loop
// Loop
for (int i = 1; i <= numberOfAttempts; i++) {
System.out.println("Attempt " + i);
}
- A for loop runs from 1 to numberOfAttempts inclusive, printing each attempt number.
Exception Handling
// Exception handling
try {
// Simulating a test step
performTestStep();
} catch (Exception e) {
System.out.println("Test step failed: " + e.getMessage());
}
- A try block is used to execute code that might throw an exception. It calls the performTestStep method. If an exception is thrown, the catch block catches it and prints an error message.
Helper Method
private static void performTestStep() throws Exception {
// Simulating a test step that might throw an exception
double randomValue = Math.random();
if (randomValue < 0.5) {
throw new Exception("Random failure occurred");
}
}
‘performTestStep’ is a private static method that simulates a test step. It generates a random value between 0 and 1. If the random value is less than 0.5, it throws an exception with the message “Random failure occurred”.
Explanation
- This code defines a basic framework for a QA automation test.
- It includes initializing variables, using control structures (if-else), loops (for), and exception handling (try-catch).
- It simulates running a test with multiple attempts and handling potential errors during test steps.
Pro tip: Don’t rush this stage! A solid foundation will pay dividends as you progress to more advanced topics.
Don’t underestimate the power of mastering the basics. I’ve seen many testers rush into advanced frameworks without a solid grasp of Java fundamentals. Those who took the time to really understand core concepts like OOP and exception handling were able to write more robust, efficient test scripts in the long run. It’s worth the initial investment.
3. Dive into Automation-Specific Java Libraries
Once you’ve got the basics down, it’s time to explore libraries that are crucial for QA automation:
– Selenium WebDriver: The gold standard for web application testing
– TestNG: A powerful testing framework inspired by JUnit and NUnit
– REST Assured: Simplifies API testing with a domain-specific language
Action item: Create a small project using each of these libraries to gain hands-on experience.
Below, I’ll outline how to create small projects using Selenium WebDriver, TestNG, and REST Assured to gain hands-on experience with each library.
Selenium WebDriver Project
Prerequisites:
- Install Java Development Kit (JDK)
- Install an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse
- Add Selenium WebDriver dependencies
Project Setup:
Create a New Maven Project:
<!-- pom.xml -->
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>selenium-example</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.0.0</version>
</dependency>
</dependencies>
</project>
Create a Test Class:
// src/main/java/com/example/SeleniumTest.java
package com.example;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
public class SeleniumTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
String title = driver.getTitle();
System.out.println("Page title is: " + title);
driver.findElement(By.id("exampleId")).click();
driver.quit();
}
}
Run the Test:
- Execute the SeleniumTest class to run your Selenium WebDriver test.
TestNG Project
Prerequisites:
- Install JDK
- Install an IDE
- Add TestNG dependencies
Project Setup:
Create a New Maven Project:
<!-- pom.xml -->
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>testng-example</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.4.0</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Create a Test Class:
// src/test/java/com/example/TestNGTest.java
package com.example;
import org.testng.annotations.Test;
import org.testng.Assert;
public class TestNGTest {
@Test
public void testAddition() {
int a = 5;
int b = 3;
int sum = a + b;
Assert.assertEquals(sum, 8);
}
}
Run the Test:
- Execute the TestNGTest class using the TestNG plugin in your IDE or by running mvn test from the command line.
REST Assured Project
Prerequisites:
- Install JDK
- Install an IDE
- Add REST Assured dependencies
Project Setup:
Create a New Maven Project:
<!-- pom.xml -->
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>restassured-example</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>4.3.3</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Create a Test Class:
// src/test/java/com/example/RestAssuredTest.java
package com.example;
import io.restassured.RestAssured;
import io.restassured.response.Response;
import org.testng.Assert;
import org.testng.annotations.Test;
public class RestAssuredTest {
@Test
public void testGetRequest() {
Response response = RestAssured.get("https://jsonplaceholder.typicode.com/posts/1");
Assert.assertEquals(response.getStatusCode(), 200);
System.out.println(response.getBody().asString());
}
}
Run the Test:
- Execute the RestAssuredTest class using the TestNG plugin in your IDE or by running mvn test from the command line.
Explanation
Each of these projects demonstrates basic usage of Selenium WebDriver, TestNG, and REST Assured. You can expand upon these examples to create more complex tests and integrate them into larger automation frameworks.
Sample Projects with Selenium WebDriver, TestNG, and REST Assured
Selenium WebDriver Project
Prerequisites:
- Java Development Kit (JDK)
- IDE (IntelliJ IDEA, Eclipse, etc.)
- Selenium WebDriver Dependencies
Project Setup:
Create a New Maven Project:
<!-- pom.xml -->
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>selenium-example</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.0.0</version>
</dependency>
</dependencies>
</project>
Create a Test Class:
// src/main/java/com/example/SeleniumTest.java
package com.example;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
public class SeleniumTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
String title = driver.getTitle();
System.out.println("Page title is: " + title);
driver.findElement(By.id("exampleId")).click();
driver.quit();
}
}
Run the Test:
- Execute the SeleniumTest class to run your Selenium WebDriver test.
Explanation:
- Create a Maven project to manage dependencies.
- Set up WebDriver to automate browser interactions.
- Navigate to a URL and perform actions (like clicking elements).
- Expand by adding more tests, using different browsers, or integrating with TestNG.
TestNG Project
Prerequisites:
- JDK
- IDE
- TestNG Dependencies
Project Setup
Create a New Maven Project:
<!-- pom.xml -->
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>testng-example</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.4.0</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Create a Test Class:
// src/test/java/com/example/TestNGTest.java
package com.example;
import org.testng.annotations.Test;
import org.testng.Assert;
public class TestNGTest {
@Test
public void testAddition() {
int a = 5;
int b = 3;
int sum = a + b;
Assert.assertEquals(sum, 8);
}
}
Run the Test:
Execute the TestNGTest class using the TestNG plugin in your IDE or by running mvn test from the command line.
Explanation:
- Create a Maven project to manage dependencies.
- Write TestNG tests to verify code functionality.
- Run tests with TestNG in IDE or via Maven.
- Expand by adding more test cases, using data providers, or integrating with Selenium.
REST Assured Project
Prerequisites:
- JDK
- IDE
- REST Assured Dependencies
Project Setup:
Create a New Maven Project:
<!-- pom.xml -->
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>restassured-example</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>4.3.3</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Create a Test Class:
// src/test/java/com/example/RestAssuredTest.java
package com.example;
import io.restassured.RestAssured;
import io.restassured.response.Response;
import org.testng.Assert;
import org.testng.annotations.Test;
public class RestAssuredTest {
@Test
public void testGetRequest() {
Response response = RestAssured.get("https://jsonplaceholder.typicode.com/posts/1");
Assert.assertEquals(response.getStatusCode(), 200);
System.out.println(response.getBody().asString());
}
}
Run the Test:
Execute the RestAssuredTest class using the TestNG plugin in your IDE or by running mvn test from the command line.
Explanation:
- Create a Maven project to manage dependencies.
- Write REST Assured tests to validate API responses.
- Run tests with TestNG in IDE or via Maven.
- Expand by adding more endpoints, request types (POST, PUT, DELETE), or integrating with CI/CD pipelines.
Explanation
- Selenium WebDriver: Automates browser interactions.
- TestNG: Organizes and runs tests.
- REST Assured: Simplifies API testing.
- Expand projects by adding more tests, integrating frameworks, or incorporating continuous integration tools.
Let’s expand the projects by integrating frameworks for the 3 above
To expand the projects and integrate them into a more robust framework, we will create a unified structure that incorporates Selenium WebDriver for UI testing, TestNG for test organization and execution, and REST Assured for API testing. We’ll also set up Maven to manage dependencies and potentially integrate with a CI/CD tool like Jenkins for continuous testing.
Unified Project Setup
Prerequisites:
- Java Development Kit (JDK)
- Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse
- Maven
- ChromeDriver (or another WebDriver)
- Jenkins (optional for CI/CD integration)
Project Structure
automation-framework
|-- src
| |-- main
| | |-- java
| | | |-- com
| | | | |-- example
| | | | | |-- tests
| | | | | | |-- SeleniumTests.java
| | | | | | |-- ApiTests.java
| | | | | |-- utils
| | | | | | |-- WebDriverSetup.java
| | |-- resources
| |-- test
| |-- java
| | |-- com
| | | |-- example
| | | | |-- TestSuite.java
|-- pom.xml
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>automation-framework</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.0.0</version>
</dependency>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.4.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>4.3.3</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
<configuration>
<suiteXmlFiles>
<suiteXmlFile>src/test/resources/testng.xml</suiteXmlFile>
</suiteXmlFiles>
</configuration>
</plugin>
</plugins>
</build>
</project>
WebDriverSetup.java
package com.example.utils;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class WebDriverSetup {
public static WebDriver getDriver() {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
return new ChromeDriver();
}
}
SeleniumTests.java
package com.example.tests;
import com.example.utils.WebDriverSetup;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.By;
import org.testng.annotations.AfterMethod;
import org.testng.annotations.BeforeMethod;
import org.testng.annotations.Test;
import static org.testng.Assert.assertEquals;
public class SeleniumTests {
private WebDriver driver;
@BeforeMethod
public void setUp() {
driver = WebDriverSetup.getDriver();
}
@Test
public void testTitle() {
driver.get("http://example.com");
String title = driver.getTitle();
assertEquals(title, "Example Domain");
}
@Test
public void testClick() {
driver.get("http://example.com");
driver.findElement(By.id("exampleId")).click();
}
@AfterMethod
public void tearDown() {
if (driver != null) {
driver.quit();
}
}
}
ApiTests.java
package com.example.tests;
import io.restassured.RestAssured;
import io.restassured.response.Response;
import org.testng.annotations.Test;
import static org.testng.Assert.assertEquals;
public class ApiTests {
@Test
public void testGetRequest() {
Response response = RestAssured.get("https://jsonplaceholder.typicode.com/posts/1");
assertEquals(response.getStatusCode(), 200);
System.out.println(response.getBody().asString());
}
}
TestSuite.java
package com.example;
import org.testng.annotations.Test;
@Test
public class TestSuite {
// This class can be used to group tests if needed
}
testng.xml
<!DOCTYPE suite SYSTEM "https://testng.org/testng-1.0.dtd" >
<suite name="Automation Test Suite">
<test name="Selenium Tests">
<classes>
<class name="com.example.tests.SeleniumTests"/>
</classes>
</test>
<test name="API Tests">
<classes>
<class name="com.example.tests.ApiTests"/>
</classes>
</test>
</suite>
Explanation:
Selenium WebDriver Integration:
- WebDriverSetup.java: Centralizes WebDriver setup for reuse across tests.
- SeleniumTests.java: Contains tests for UI interactions.
TestNG Integration:
- testng.xml: Configures TestNG to run tests.
- TestSuite.java: (Optional) groups tests logically if needed.
REST Assured Integration:
- ApiTests.java: Contains tests for API interactions.
Maven: Manages dependencies and builds the project.
- Surefire Plugin: Integrates TestNG with Maven.
CI/CD Integration (Optional):
- Jenkins: Configure a Jenkins job to run mvn test to execute tests on each commit or periodically.
Running Tests
- Use Maven to run tests:
mvn test
Expanding the Framework:
- Page Object Model (POM): For more complex UI tests, implement the POM design pattern.
- Parameterized Tests: Use TestNG data providers for parameterized tests.
- CI/CD: Integrate with Jenkins to run tests automatically.
- Reporting: Add reporting tools like Allure or ExtentReports.
- Cross-Browser Testing: Extend WebDriver setup for different browsers.
This unified approach integrates Selenium WebDriver for UI testing, TestNG for test organization and execution, and REST Assured for API testing, providing a comprehensive automation framework.
Success Story: From Manual Tester to Automation Expert
When Alex Patel started working as a junior manual tester, he had no programming experience. “I was intimidated by the thought of learning to code,” Alex recalls. “But our lead encouraged me to start with Selenium WebDriver and Java.”
Alex started small, automating a few simple test cases. As he grew more confident, he expanded his skills to include TestNG for test management and REST Assured for API testing.
“Within a year, I had automated our entire regression suite,” Alex says proudly. “We reduced our regression testing time from two weeks to just two days. It was a huge win for the team.”
Today, Alex leads automation efforts. His advice to newcomers? “Don’t be afraid to start small. Each library you learn builds on the last. Before you know it, you’ll be creating powerful, efficient test frameworks that make a real difference to your team.”
4. Embrace Design Patterns and Best Practices
Elevate your code from functional to professional by implementing design patterns and best practices:
– Page Object Model (POM) for web automation
– Data-driven testing techniques
– Singleton pattern for managing WebDriver instances
Remember: Clean, maintainable code is the hallmark of a top-tier QA automation engineer.
5. Continuous Learning: Stay Updated with Java Trends
The tech world moves fast, and Java is no exception. Stay ahead of the curve by:
– Following Java blogs and newsletters
– Participating in online Java communities (Stack Overflow, Reddit r/java)
– Attending virtual Java conferences and webinars
Did you know? Java releases new features every six months. Staying updated ensures you’re always using the latest and greatest tools.
6. Practice, Practice, Practice: Real-World Projects
Theory is important, but nothing beats hands-on experience. Challenge yourself with:
– Open-source projects on GitHub
– Personal automation projects (e.g., automating your favorite website)
– Contributing to existing test automation frameworks
Theory is important, but the real learning happens when you’re knee-deep in a project. I always tell my mentees to find an open-source project they’re passionate about and start contributing. It’s the fastest way to improve your skills and build a portfolio that will impress potential employers.
Bonus tip: Document your projects and share them on platforms like LinkedIn to showcase your skills to potential employers.
7. Certifications: Validate Your Java Expertise
While not mandatory, certifications can give you an edge in the job market. Consider pursuing:
– Oracle Certified Professional: Java SE 11 Developer
– ISTQB Certified Tester Foundation Level
Mastering Java for QA automation was the best career decision I ever made. It not only made me more efficient in my day-to-day work but opened doors I never thought possible. I’ve gone from being just another tester to a sought-after automation architect. Companies are always on the lookout for QA pros who can code, and Java skills put you at the top of that list.
Frequently Asked Questions
Q1: How long does it take to learn Java for QA automation?
A: The learning curve varies, but with dedicated study and practice, you can become proficient in 3-6 months. Remember, learning is a continuous process in tech!
Q2: Do I need a computer science degree to learn Java for QA?
A: While a CS degree can be helpful, it’s not mandatory. Many successful QA automation engineers are self-taught or come from bootcamps.
Q3: What’s the best way to practice Java for QA automation?
A: Start by automating simple web applications, then progress to more complex scenarios. Open-source projects and online coding challenges are great resources.
Q4: Is Java the only language for QA automation?
A: No, but it’s one of the most popular. Python and JavaScript are also widely used in QA automation.
Q5: How can I stay motivated while learning Java?
A: Set small, achievable goals, join online communities for support, and celebrate your progress.
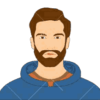
Tony, a revered technical author and expert in software testing and quality assurance, has his roots in Texas. He embarked on his professional journey at Caltech, diving deep into Informatics. This laid a robust academic groundwork, further cultivating his analytical prowess and understanding of software testing and quality assurance principles.
Post-graduation, Tony’s career took flight at GE. As a Senior QA professional, he was integral in maintaining software system reliability and performance. His intricate strategies facilitated numerous project successes.
Craving new challenges, Tony then moved to eBay. Here, his ability to identify risks and execute effective testing processes ensured seamless user experiences. Subsequently, he joined Uber, ensuring the safety and reliability of the company’s software platforms through efficient testing frameworks.
Despite professional feats, Tony enjoys a grounded personal life in Texas, nurturing his two sons and indulging in local cultural events. As a technical author, his insightful writings have helped shape the industry, guiding countless professionals. Tony’s enduring dedication and expertise continue to influence software testing and quality assurance, marking him as a true pioneer in the field.