Functional testing is a critical component of e-commerce website quality assurance. It helps identify bugs and issues that could negatively impact the customer experience. Drawing from my years of experience in the e-commerce industry, I’ve crafted a functional testing method that integrates the precision of automation with the insight of human observation.
Why Functional Testing is Essential for E-commerce Owners
Functional testing is crucial for e-commerce owners because it ensures that your website works as intended, providing a smooth and satisfying experience for customers. Here are key reasons why it’s important:
User Experience
Functional testing checks that every part of your website operates correctly. For instance, testing the registration process ensures users can sign up without issues. A seamless experience keeps customers happy and encourages repeat visits.
Securing Transactions
Functional testing ensures secure payment processing. It verifies that payment gateways handle transactions safely and that customers receive order confirmations. This builds trust and protects your business from fraud.
Optimizing Performance
Functional testing helps identify and fix performance issues. By simulating heavy traffic, it ensures your site can handle peak shopping times without slowing down or crashing. This prevents lost sales during high-demand periods.
Mobile Responsiveness
With many customers shopping via mobile devices, functional testing verifies that your site works well on all screen sizes. This broadens your reach and improves accessibility, catering to a larger audience.
Reducing Costs
By catching defects early, functional testing saves money. It prevents costly fixes after the site is live and reduces downtime. This efficiency allows for faster updates and improvements.
Improving SEO
Functional testing enhances SEO by ensuring fast load times, mobile responsiveness, and overall site integrity. Better SEO attracts more traffic, increasing visibility and sales.
Preventing Revenue Loss
Functional testing ensures critical features like the shopping cart and checkout process work perfectly. This prevents abandoned carts and lost sales, directly impacting your revenue.
Building Brand Reputation
A well-functioning website builds trust and a strong brand reputation. Customers are more likely to return and recommend your site if they have a positive experience. Functional testing helps maintain this standard.
Here are some key aspects of functional testing for e-commerce sites:
User Registration and Login
Testing the user registration and login functionalities is crucial as these are the first points of interaction for new customers. The process must be smooth and error-free to ensure a positive initial experience.
Example: Testing User Registration and Login
Automated Test Script Using Selenium WebDriver
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
driver = webdriver.Chrome()
# Test user registration
driver.get("https://example-ecommerce.com/register")
driver.find_element_by_name("username").send_keys("testuser")
driver.find_element_by_name("email").send_keys("testuser@example.com")
driver.find_element_by_name("password").send_keys("Password123")
driver.find_element_by_name("confirm_password").send_keys("Password123")
driver.find_element_by_name("submit").click()
assert "Registration successful" in driver.page_source
# Test user login
driver.get("https://example-ecommerce.com/login")
driver.find_element_by_name("username").send_keys("testuser")
driver.find_element_by_name("password").send_keys("Password123")
driver.find_element_by_name("submit").click()
assert "Welcome, testuser" in driver.page_source
driver.quit()
This script automates the registration and login process, ensuring that new users can create an account and log in without issues. Additionally, manual testing should verify the user experience, such as error messages for invalid inputs and the ease of navigating between registration and login pages.
Product Search and Navigation
A well-functioning search and navigation system is vital for users to find products quickly and efficiently. Functional testing should ensure that search results are accurate, filters work correctly, and categories and menus are fully operational.
Example: Testing Product Search and Navigation
Automated Test Script Using Selenium WebDriver
from selenium import webdriver
driver = webdriver.Chrome()
# Test product search
driver.get("https://example-ecommerce.com")
driver.find_element_by_name("search").send_keys("laptop")
driver.find_element_by_name("search_button").click()
assert "Search results for 'laptop'" in driver.page_source
assert driver.find_elements_by_class_name("product-item")
# Test category navigation
driver.find_element_by_link_text("Electronics").click()
assert "Electronics" in driver.page_source
assert driver.find_elements_by_class_name("product-item")
driver.quit()
This script tests the search functionality by searching for a product and verifying the presence of search results. It also tests the navigation by clicking on a category link and checking if the products in that category are displayed. Manual testing can complement this by ensuring the filters and sorting options work correctly and the navigation is intuitive.
Product Pages
Product pages must display accurate information, including product descriptions, images, pricing, and availability. Functional testing should verify that all elements on the product page are correct and consistent.
Example: Testing Product Pages
Automated Test Script Using Selenium WebDriver
from selenium import webdriver
driver = webdriver.Chrome()
# Test product page
driver.get("https://example-ecommerce.com/product/laptop")
assert "Laptop" in driver.page_source
assert driver.find_element_by_id("product-title").text == "Laptop"
assert driver.find_element_by_id("product-price").text == "$999.99"
assert driver.find_element_by_id("product-availability").text == "In Stock"
driver.quit()
This script verifies that the product page displays the correct title, price, and availability status. Manual testing should ensure that images load correctly, and additional information such as reviews and specifications are accurate.
Shopping Cart
The shopping cart functionality is central to the e-commerce experience. It should allow users to add, remove, and update item quantities seamlessly. Testing should also ensure that promotional codes are applied correctly.
Example: Testing Shopping Cart Functionality
Automated Test Script Using Selenium WebDriver
from selenium import webdriver
driver = webdriver.Chrome()
# Test adding item to cart
driver.get("https://example-ecommerce.com/product/laptop")
driver.find_element_by_id("add-to-cart").click()
assert "Item added to cart" in driver.page_source
# Test viewing cart
driver.get("https://example-ecommerce.com/cart")
assert "Laptop" in driver.page_source
assert driver.find_element_by_id("cart-quantity").get_attribute("value") == "1"
# Test updating quantity
driver.find_element_by_id("cart-quantity").clear()
driver.find_element_by_id("cart-quantity").send_keys("2")
driver.find_element_by_id("update-cart").click()
assert driver.find_element_by_id("cart-quantity").get_attribute("value") == "2"
# Test applying promo code
driver.find_element_by_id("promo-code").send_keys("DISCOUNT10")
driver.find_element_by_id("apply-promo").click()
assert "Promo code applied" in driver.page_source
driver.quit()
This script tests the shopping cart by adding an item, viewing the cart, updating the item quantity, and applying a promo code. Manual testing should also check for any edge cases, such as removing items and verifying cart totals.
Checkout Process
The checkout process is the final step before a purchase is completed. It must be smooth and error-free to ensure conversions. Testing should verify each step of the checkout flow, including address entry, shipping options, and order review.
Example: Testing Checkout Process
Automated Test Script Using Selenium WebDriver
from selenium import webdriver
driver = webdriver.Chrome()
# Test checkout process
driver.get("https://example-ecommerce.com/cart")
driver.find_element_by_id("checkout").click()
# Enter address details
driver.find_element_by_id("address-line1").send_keys("123 Main St")
driver.find_element_by_id("city").send_keys("Testville")
driver.find_element_by_id("zip").send_keys("12345")
driver.find_element_by_id("country").send_keys("USA")
driver.find_element_by_id("continue").click()
# Select shipping option
driver.find_element_by_id("shipping-option-standard").click()
driver.find_element_by_id("continue").click()
# Review order
assert "Order Review" in driver.page_source
assert "123 Main St" in driver.page_source
assert "Standard Shipping" in driver.page_source
driver.quit()
This script tests the checkout process from the cart to the order review stage. Manual testing should also cover edge cases like incomplete address information, different shipping options, and ensuring that users can go back and edit information without losing data.
Payment Processing
Testing payment processing is crucial as it involves handling sensitive financial information. Various payment methods should be tested to ensure transactions are processed securely and order confirmations are sent.
Example: Testing Payment Processing
Automated Test Script Using Selenium WebDriver
from selenium import webdriver
driver = webdriver.Chrome()
# Test payment processing
driver.get("https://example-ecommerce.com/checkout")
driver.find_element_by_id("payment-method-credit-card").click()
driver.find_element_by_id("card-number").send_keys("4111111111111111")
driver.find_element_by_id("expiry-date").send_keys("12/23")
driver.find_element_by_id("cvv").send_keys("123")
driver.find_element_by_id("complete-purchase").click()
assert "Order Confirmation" in driver.page_source
assert "Thank you for your purchase" in driver.page_source
driver.quit()
This script tests the payment processing using a test credit card number. Manual testing should verify the handling of various payment methods, such as PayPal or gift cards, and ensure secure transmission of payment data.
Order Management
Once an order is placed, it needs to be correctly recorded in the backend system and trackable by customers. Testing should verify that orders are accurately captured and customers can view their order status.
Example: Testing Order Management
Automated Test Script Using Selenium WebDriver
from selenium import webdriver
driver = webdriver.Chrome()
# Test order tracking
driver.get("https://example-ecommerce.com/account/orders")
assert "Order #12345" in driver.page_source
assert "Shipped" in driver.page_source
driver.quit()
This script tests that orders appear correctly in the user’s account and that the status is updated. Manual testing should also verify the accuracy of order details and the ability to handle order modifications, such as cancellations or returns.
Promotions and Discounts
Promotional offers and discount codes are common in e-commerce. Testing should ensure these are applied correctly and reflected in the cart totals and order summaries.
Example: Testing Promotions and Discounts
Automated Test Script Using Selenium WebDriver
from selenium import webdriver
driver = webdriver.Chrome()
# Test applying discount code
driver.get("https://example-ecommerce.com/cart")
driver.find_element_by_id("promo-code").send_keys("DISCOUNT10")
driver.find_element_by_id("apply-promo").click()
assert "Promo code applied" in driver.page_source
assert "$10.00 discount" in driver.page_source
driver.quit()
This script tests the application of a discount code and verifies that the discount is reflected in the cart total. Manual testing should also check for edge cases, such as expired or invalid promo codes.
User Reviews and Ratings
Allowing customers to leave reviews and ratings enhances trust and provides valuable feedback. Testing should verify that this functionality works correctly.
Example: Testing User Reviews and Ratings
Automated Test Script Using Selenium WebDriver
from selenium import webdriver
driver = webdriver.Chrome()
# Test leaving a review
driver.get("https://example-ecommerce.com/product/laptop")
driver.find_element_by_id("write-review").click()
driver.find_element_by_id("review-text").send_keys("Great laptop!")
driver.find_element_by_id("review-rating").send_keys("5")
driver.find_element_by_id("submit-review").click()
assert "Review submitted" in driver.page_source
assert "Great laptop!" in driver.page_source
driver.quit()
This script tests the process of leaving a product review and rating. Manual testing should ensure the moderation of reviews, if applicable, and verify the display of reviews on the product page.
Wishlist Functionality
Wishlist functionality allows customers to save items for later. Testing should ensure users can add and remove items from their wishlists seamlessly.
Example: Testing Wishlist Functionality
Automated Test Script Using Selenium WebDriver
from selenium import webdriver
driver = webdriver.Chrome()
# Test adding item to wishlist
driver.get("https://example-ecommerce.com/product/laptop")
driver.find_element_by_id("add-to-wishlist").click()
assert "Item added to wishlist" in driver.page_source
# Test viewing wishlist
driver.get("https://example-ecommerce.com/wishlist")
assert "Laptop" in driver.page_source
driver.quit()
This script tests adding an item to the wishlist and viewing the wishlist. Manual testing should verify that items can be easily removed and that the wishlist persists across sessions.
Mobile Responsiveness
With the increasing use of mobile devices for online shopping, ensuring mobile responsiveness is crucial. Testing should verify the site’s functionality across different devices and screen sizes.
Example: Testing Mobile Responsiveness
Using BrowserStack for Mobile Testing
Sign up for BrowserStack:
Create an account at BrowserStack.
Configure and run tests on various devices:
Use BrowserStack to test the site on different mobile devices and screen sizes.
from selenium import webdriver
desired_cap = {
'browser': 'Chrome',
'browser_version': 'latest',
'os': 'Windows',
'os_version': '10',
'resolution': '1024x768',
'name': 'Bstack-[Python] Sample Test'
}
driver = webdriver.Remote(
command_executor='https://YOUR_USERNAME:YOUR_ACCESS_KEY@hub-cloud.browserstack.com/wd/hub',
desired_capabilities=desired_cap
)
driver.get("https://example-ecommerce.com")
# Simulate mobile view
driver.set_window_size(375, 667) # iPhone 6/7/8 dimensions
assert "E-commerce" in driver.title
driver.quit()
By using BrowserStack, QA testers can ensure that the site functions properly across a variety of devices, providing a consistent user experience regardless of the device used.
Cross-browser Compatibility
Testing across various web browsers ensures consistent functionality and appearance. This is crucial as users may access the site using different browsers.
Example: Cross-browser Testing Using Selenium Grid
- Set up Selenium Grid:
- Install and configure the Selenium Grid hub and nodes.
- Launch the hub and register the nodes to it.
# Start the hub
java -jar selenium-server-standalone.jar -role hub
# Start a node and register to the hub
java -jar selenium-server-standalone.jar -role node -hub http://localhost:4444/grid/register
Create a Test Script:
- Write a Selenium test script to check the website’s functionality on different browsers.
from selenium import webdriver
from selenium.webdriver.common.desired_capabilities import DesiredCapabilities
# Set up desired capabilities for different browsers
browsers = ['chrome', 'firefox', 'safari', 'edge']
for browser in browsers:
driver = webdriver.Remote(
command_executor='http://localhost:4444/wd/hub',
desired_capabilities=getattr(DesiredCapabilities, browser.upper())
)
driver.get("https://example-ecommerce.com")
# Perform test actions
assert "Example E-commerce" in driver.title
driver.find_element_by_id("add-to-cart-button").click()
assert "Cart" in driver.title
driver.quit()
By running tests across various browsers, QA ensures that the e-commerce platform provides a consistent user experience, regardless of the browser or device used.
Wrapping up
Functional testing is an essential part of ensuring a seamless and enjoyable user experience on e-commerce platforms. By meticulously testing key aspects such as user registration, product search, shopping cart functionality, checkout processes, payment processing, and more, businesses can identify and rectify issues before they impact customers. This not only enhances customer satisfaction but also boosts conversions and brand loyalty.
For competent and reliable QA services tailored to your e-commerce needs, consider partnering with QATPRO. Our experienced team of professionals and cost-effective solutions can help you maintain the highest quality standards for your platform, ensuring a flawless user experience and securing your position in the competitive e-commerce market.
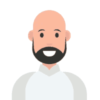
Joshua, a distinguished technical author and expert in software testing and quality assurance, originated from the dynamic city of London. His journey began at Imperial College London, where his fascination with technology bloomed into a profound understanding of software intricacies.
After graduation, Joshua carved his path at UNESCO, overseeing quality assurance teams and processes with a keen eye for detail. His career then took him to Microsoft and Twitter, where he honed his skills further, leading developments and implementing testing strategies. His efforts were central to enhancing user experiences, as he championed efficiency and innovative methodologies.
Beyond his professional role, Joshua’s dedication extended to educating others. His technical writings, appreciated for their clarity and depth, have significantly influenced the industry.
Despite his remarkable professional achievements, Joshua maintains a balanced life in London, enjoying tranquil retreats with his wife, Tracy, and drawing inspiration from their adventures. His unyielding commitment to excellence in software testing is shaping the technological landscape for future generations.